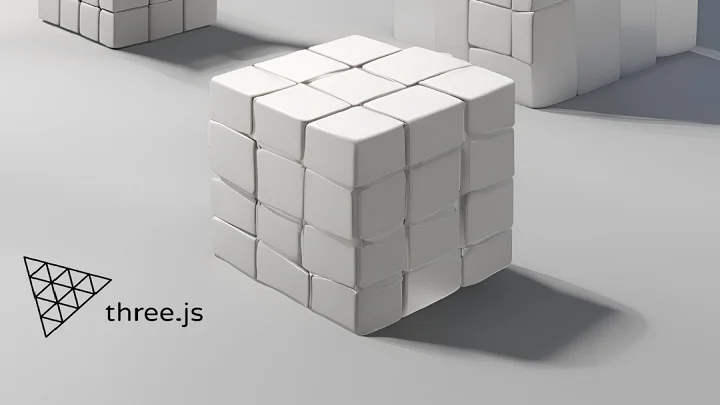
Getting Started with MeshBasicMaterial in Three.js:A Step-by-Step Guide
MeshBasicMaterial
is a crucial component in Three.js that allows developers to create impressive 3D visualizations without considering environmental lighting. This material is perfect for objects that should maintain their appearance regardless of ambient lighting.
Want to take your Three.js skills to the next level? Explore our comprehensive roadmap of Three.js courses and start building stunning 3D visualizations today! Three.js roadmap
Setting Up Your Scene
Before working with MeshBasicMaterial
, ensure you have set up your basic scene in Three.js. This includes creating a camera, a renderer, and perhaps some geometric objects to apply the material to.
import * as THREE from 'three';
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls';
// Create scene
const scene = new THREE.Scene();
// Create camera
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.z = 5;
// Create renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// Add orbital controls
const controls = new OrbitControls(camera, renderer.domElement);
Configuring MeshBasicMaterial
To use MeshBasicMaterial
, you first need to instantiate the object and then assign it to your mesh. Here's how to do it step by step:
// Create a new instance of MeshBasicMaterial
const material = new THREE.MeshBasicMaterial({ color: 0xffffff });
// Assign the material to your mesh
const geometry = new THREE.BoxGeometry(1, 1, 1);
const mesh = new THREE.Mesh(geometry, material);
scene.add(mesh);
Properties of MeshBasicMaterial
MeshBasicMaterial
offers several properties that you can adjust according to your specific needs:
- color: Defines the color of the material.
- transparent: Indicates whether the material is transparent or not.
- opacity: Controls the transparency of the material.
- wireframe: Displays the model in wireframe mode.
Here's an example of how to configure these properties:
material.color.setHex(0xff0000); // Change color to red
material.transparent = true; // Make the material transparent
material.opacity = 0.5; // Set opacity to 50%
material.wireframe = true; // Enable wireframe mode
Using Textures with MeshBasicMaterial
Textures can significantly enhance the visual details of your 3D objects. Here's how to load and apply a texture using TextureLoader
:
import { TextureLoader } from 'three';
// Load a texture
const textureLoader = new TextureLoader();
const texture = textureLoader.load('path/to/your/texture.jpg');
// Assign the texture to the material
material.map = texture;
In this enhanced guide, we've covered everything from initial setup to advanced usage of MeshBasicMaterial
in Three.js. By following these steps, you'll be able to create stunning 3D visualizations using this powerful yet simple material.
Ready to take your Three.js skills to the next level? Explore our comprehensive roadmap of Three.js courses and start building impressive 3D visualizations today Three.js course guide.