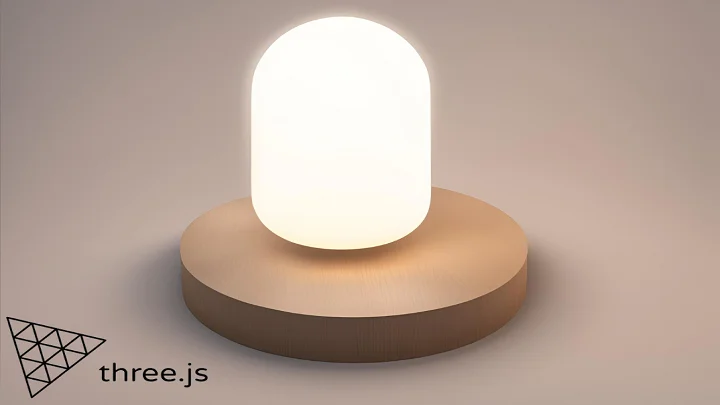
How to Use Lighting in Three.js:A Step-by-Step Guide
Lighting is a crucial aspect of creating realistic and engaging 3D experiences in Three.js. In this step-by-step guide, we will cover the basics of lighting in Three.js, including ambient, directional, and spot lights. By the end of this guide, you will have a solid understanding of how to use lighting in Three.js and be able to create stunning 3D visualizations.
Want to take your Three.js skills to the next level? Explore our comprehensive roadmap of Three.js courses and start building stunning 3D visualizations today! Three.js Roadmap
Setting Up Lighting
To start using lighting in Three.js, you need to create a new instance of the AmbientLight
class and add it to your scene.
import { AmbientLight } from 'three';
// Create a new ambient light
const light = new AmbientLight(0xffffff, 0.5);
// Add the light to the scene
scene.add(light);
Configuring Ambient Light
The ambient light has several properties that can be configured to suit your specific needs. Here are some of the most important ones:
- color: The color of the ambient light.
- intensity: The intensity of the ambient light.
Here's an example of how to configure these properties:
light.color.setHex(0xffffff); // Corrected method to set color
light.intensity = 0.5;
Setting Up Directional Light
To set up a directional light, you need to create a new instance of the DirectionalLight
class and add it to your scene.
import { DirectionalLight } from 'three';
// Create a new directional light
const light = new DirectionalLight(0xffffff, 0.5);
// Position the light
light.position.set(0, 10, 10);
// Add the light to the scene
scene.add(light);
Configuring Directional Light
The directional light has several properties that can be configured to suit your specific needs. Here are some of the most important ones:
- color: The color of the directional light.
- intensity: The intensity of the directional light.
- position: The position of the directional light.
Here's an example of how to configure these properties:
light.color.setHex(0xffffff); // Corrected method to set color
light.intensity = 0.5;
light.position.set(0, 10, 10); // Example position
Setting Up Spot Light
To set up a spot light, you need to create a new instance of the SpotLight
class and add it to your scene.
import { SpotLight } from 'three';
// Create a new spot light
const light = new SpotLight(0xffffff, 0.5, 0, Math.PI / 4, 0);
// Position the light
light.position.set(0, 10, 10);
// Add the light to the scene
scene.add(light);
Configuring Spot Light
The spot light has several properties that can be configured to suit your specific needs. Here are some of the most important ones:
- color: The color of the spot light.
- intensity: The intensity of the spot light.
- distance: The maximum distance of the light.
- angle: The angle of the cone of light.
- penumbra: The percentage of the cone that is attenuated due to penumbra.
Here's an example of how to configure these properties:
light.color.setHex(0xffffff); // Corrected method to set color
light.intensity = 0.5;
light.distance = 0; // No limit
light.angle = Math.PI / 4; // 45 degrees
light.penumbra = 0.1; // 10% penumbra
light.position.set(0, 10, 10); // Example position
In this step-by-step guide, we covered the basics of lighting in Three.js, including ambient, directional, and spot lights. By understanding how to use these types of lights, you can create realistic and engaging 3D experiences. For more advanced techniques and best practices, check out our roadmap of Three.js courses at Three.js Course.